Integrating Proxies with Axios
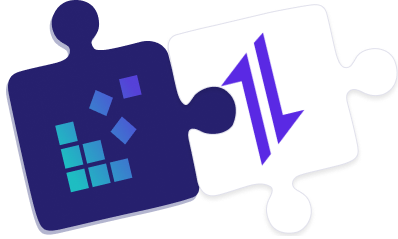
Installing Axios
To install Axios, you can use any JavaScript package manager like npm, Yarn, or Bower. For npm, you can install it by running the following command:
npm install axios
Alternatively, you can also install Axios using a CDN, as demonstrated below:
<script src=”https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js”></script>
For other packages or alternative CDNs, please consult the official documentation provided by Axios.
Setting up proxies
Let’s explore the following code snippet to set up proxies with Axios:
const browser = require('axios')
browser(
{
url: 'https://example.com',
proxy: {
protocol: 'http',
host: '<host_address>',
port: <port_number>
}
}
).then(result => {
console.log(result.data)
}).catch(error => console.error(error))
The code is quite simple. We start by creating a browser object using Axios. Then, we make a GET request to a website called example.com. When making the GET request, we include a dictionary with the proxy details. In the dictionary, we specify the protocol (like HTTP or HTTPS), the host (the proxy server address), and the port (the proxy server port number). This information helps Axios determine which proxy to use for the request.
If you want to use DataImpulse’ Residential Proxies, you need to make some changes to the code provided earlier. Here’s how you can update the information:
proxy: {
protocol: 'http',
host: 'gw.dataimpulse.com',
port: 823,
username: 'your proxy plan username',
password: 'your proxy plan password',
}
Testing proxy connection
Now, let’s test the proxy integration and verify if the connection was successful. Take a look at the code snippet below:
import { HttpsProxyAgent } from 'https-proxy-agent';
const login = "your_login_here";
const password = "your_password_here";
const browser = require('axios');
const httpsAgent = new HttpsProxyAgent(`http://${login}:${password}@gw.dataimpulse.com:823/`);
browser.get('https://ip-api.com/', { httpsAgent: httpsAgent })
.then(result => console.log(result.data))
.catch(error => console.log(error));
The provided code demonstrates the connection to a Residential Proxy.
Please remember to replace the code with your own proxy plan credentials and proxy server information.