Setting up proxies in Guzzle: PHP Tutorial
Guzzle is a PHP HTTP client designed to simplify the process of sending HTTP requests and integrating with web services. Its user-friendly interface enables the construction of query strings, handling large uploads and downloads, and more. With Guzzle, you can eliminate the need to deal with stream contexts, cURL options, or sockets.
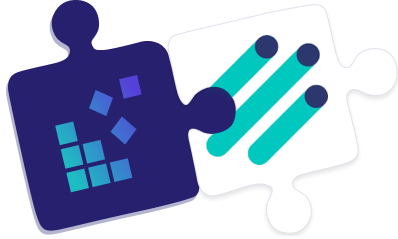
Installing Guzzle
To install Guzzle, we will utilize Composer. If you don’t have Composer installed, you can refer to the following instructions to install it.
Step 1: Installing Composer
Begin by obtaining the Composer installer using the provided command:
php -r “copy(‘https://getcomposer.org/installer’, ‘composer-setup.php’);”
Next, execute the installer by running the following command:
php composer-setup.php
Lastly, you need to move the binary to the PATH location.
sudo mv composer.phar /usr/local/bin/composer
Step 2: Installing Guzzle
Now that Composer is successfully installed, we can utilize it to install Guzzle in our PHP project. The process is straightforward; just execute the following command:
composer require guzzlehttp/guzzle
When the aforementioned command is executed, it will initiate the installation of Guzzle and its dependencies to the current working director.
Integrating Proxies
In this section, we will explore the process of integrating proxies with Guzzle.
Step 1: Begin by importing the recently installed library:
require_once 'vendor/autoload.php';
use GuzzleHttp\Client;
Step 2: Next, we can create a client object to make a GET request, as shown in the example below:
$client = new Client();
$response = $client->request('GET', 'https://ip-api.com/');
$body = $response->getBody();
echo($body);
As observed, we are creating a Client object and using it to send a GET request to the https://ip-api.com/. Additionally, we are providing the proxy as an extra argument.
Please note that in the proxy URL, we are including the username, password, IP address, and port. You should replace these values with your DataImpulse proxy plan credentials. If the IP address is public and doesn’t require any authentication, you can exclude the username and password and send the request as demonstrated below:
$client = new Client();
$response = $client->request('GET', 'https://ip-api.com/', ['proxy' => 'http://<proxy-host>:<proxy-port>']);
$body = $response->getBody();
echo($body);
Using Rotating Proxies
Utilizing rotating proxies with Guzzle is also an option. There are different methods to achieve this. For instance, if you possess a list of IP addresses, you can create an array of IPs and manually rotate through them using PHP programming. However, this can be cumbersome when dealing with a large IP list.
Alternatively, you can leverage the capabilities of DataImpulse’s solutions, which handle the rotations and proxy management automatically. In the upcoming sections, we will explore integrating DataImpulse proxies with Guzzle.
Configuring DataImpulse Residential Proxies
To begin with, let’s establish the setup for DataImpulse Residential Proxies. Specify “gw.dataimpulse.com” as the HTTP proxy server and set the port to 823. The corresponding code will appear as follows:
$client = new Client();
$response = $client->request('GET', 'http://ip-api.com/', ['proxy' => 'http://<YourProxyPlanLogin>:<YourProxyPlanPassword>@gw.dataimpulse.com:823']);
$body = $response->getBody();
echo($body);
In the provided code snippet, we retrieve the response’s body and display it using the “echo” statement. If everything functions properly, upon executing the code, you should observe an IP address in the terminal (or in the browser, depending on how you execute the PHP script).
Setting Up DataImpulse Whitelisted IP Proxy
Apart from the previously mentioned methods, DataImpulse also supports whitelisting IP addresses for specific services. Once you have whitelisted your IP from the DataImpulse dashboard, you can utilize proxies without including credentials in each request. This simplifies the setup process and enhances proxy security, as you no longer need to store your credentials in your scripts.
For instance, if you have whitelisted your IP address for using DataImpulse Residential Proxies, the previously written code can be simplified as follows:
$client = new Client();
$response = $client->request('GET', 'http://ip-api.com/', ['proxy' => 'http://gw.dataimpulse.com:823']);
$body = $response->getBody();
echo($body)
DataImpulse will automatically identify the IP address from your request and compare it with the whitelisted IP addresses. If there’s a match, your request will be granted access without requiring the inclusion of username and password credentials. Once whitelisted, you can exclude the proxy plan username and password from the code.