Integrating Proxies with Python Requests
The Python Requests library is widely recognized as a user-friendly and widely-used tool for handling HTTP/1.1 requests. With millions of monthly downloads, it has become a popular choice among developers. The library streamlines the management of HTTP requests and responses, eliminating the need for manual input of query strings in the URL.
Integrating proxies with scraping or web requests libraries is essential. By incorporating proxies, you can prevent unwanted IP blocks from target websites and reduce the risks associated with exposing your own IP address.
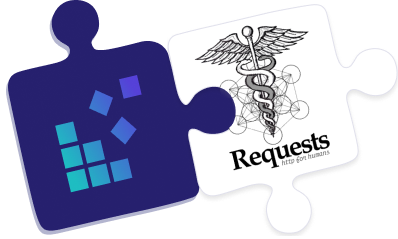
Installing Requests is simple. Below is an instruction of how you can integrate proxies into your code.
import requests
# URL to scrape
url = 'https://www.example.com' # Replace with the desired website URL
# Proxy configuration with login and password
proxy_host = 'gw.dataimpulse.com'
proxy_port = 823
proxy_login = ''
proxy_password = ''
proxy = f'http://{proxy_login}:{proxy_password}@{proxy_host}:{proxy_port}'
proxies = {
'http': proxy,
'https': proxy
}
# Send a GET request using the proxy
response = requests.get(url, proxies=proxies)
# Check if the request was successful
if response.status_code == 200:
# Process the response content
print(response.text)
else:
print('Request failed with status code:', response.status_code)
NOTE: Please replace and with your actual credentials for the proxy server so that the code works with your own proxy login and password.
That’s it! Incorporating proxies into scraping or request libraries is essential. By setting up proxies in Python Requests, you can confidently embark on your web scraping projects without concerns about IP blocks or geographical restrictions.